React JS is a powerful JavaScript library that helps in building web applications. Today we will discuss about multi-condition handling in React that how we can add multi condition in react.js. By using multi-condition in React we can handle multiple conditions without making the code messy. In this blog, we will understand how multi-conditions are used in React JS, and how it improves the performance and readability of your app.
Why does React JS Includes Multi Condition?
Whenever we are creating any complex React applications, we have to handle multiple conditions, like User authentication, status Data loading, state Error handling, UI rendering based on different user actions
If we handle all these React JS Includes Multi Condition in a simple way, then the code can become complicated. So there is an efficient and clean way of handling multi-conditions in React which also enhances performance.
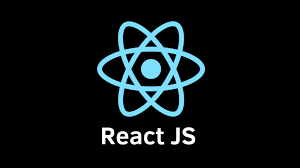
Multi-Condition Handling in React JS
To handle multi-conditions in React, we need to understand some commonly used techniques and patterns.
Ternary operator
The ternary operator is a simple way to check conditions in React. This is very useful to handle conditional rendering.
import React, { useState } from "react";
function UserGreeting() {
const [isLoggedIn, setIsLoggedIn] = useState(false);
return (
<div>
{isLoggedIn ? (
<h1>Welcome Back, User!</h1>
) : (
<h1>Please Log In</h1>
)}
</div>
);
}
export default UserGreeting;
In the above example, we are using isLoggedIn state. If the user is logged-in, then “Welcome Back, User!” will show, and if logged-out, then “Please Log In” message will appear. And its better example of React JS Includes Multi Condition
Logical AND (&&
) Operator
If you need to check a condition and then render a specific component, you can use the && operator.
import React, { useState } from "react";
function Greeting() {
const [isAdmin, setIsAdmin] = useState(false);
return (
<div>
<h1>Welcome!</h1>
{isAdmin && <p>You have admin privileges.</p>}
</div>
);
}
export default Greeting;
In the above code only if admin is true, then “You have admin privileges” will be rendered. If it is false, nothing will be visible. And its better example of React JS Includes Multi Condition
Switch-Case for Multiple Conditions
If you want to handle multiple conditions use of switch-case statement can be very helpful. This approach maintains readability and makes the code structured.
import React, { useState } from "react";
function RoleBasedContent() {
const [role, setRole] = useState("guest");
const renderContent = () => {
switch (role) {
case "admin":
return <p>Welcome, Admin!</p>;
case "user":
return <p>Welcome, User!</p>;
case "guest":
return <p>Welcome, Guest!</p>;
default:
return <p>Please log in.</p>;
}
};
return (
<div>
<h1>Role-based Content</h1>
{renderContent()}
</div>
);
}
export default RoleBasedContent;
In the above example, by using switch-case, we are rendering content on the basis of role. If the role is admin, then admin content will be visible, and if the role is guest, then guest content will be visible. And its better example of React JS Includes Multi Condition
Nested Ternary Operators
If you want to check more than one condition in a single line. In this case, a nested ternary operator is used.
import React, { useState } from "react";
function UserProfile() {
const [status, setStatus] = useState("loading");
return (
<div>
{status === "loading" ? (
<p>Loading...</p>
) : status === "error" ? (
<p>There was an error loading the data.</p>
) : (
<p>Data Loaded Successfully!</p>
)}
</div>
);
}
export default UserProfile;
In the abobe code we have checked 3 different conditions depending on the status: loading, error, and success. By using nested ternary operators we can check conditions in one line. And its better example of React JS Includes Multi Condition
Using Functions for Complex Logic
If the conditions are more complex and it is important to maintain readability, you can create a function that handles the conditions. This makes the code more clean and maintainable.
import React, { useState } from "react";
function UserStatus() {
const [status, setStatus] = useState("offline");
const getUserMessage = (status) => {
if (status === "online") {
return "You are online!";
} else if (status === "offline") {
return "You are offline.";
} else if (status === "busy") {
return "You are currently busy.";
} else {
return "Status unknown.";
}
};
return (
<div>
<h1>{getUserMessage(status)}</h1>
</div>
);
}
export default UserStatus;
In the above example, we are handling complex logic through the getSermMessage function. This approach makes the code more scalable and scalable. And its better example of React JS Includes Multi Condition
Benefits of React JS Includes Multi Condition
Code Readability:
Multi-condition handling will make your code quite readable. It is important to handle situations efficiently in React, especially when multiple cases are involved.
Optimization:
If you handle conditions efficiently, you can make your React app more performant. The clarity and simplicity of the code also makes debugging easy.
Scalability:
When new features are added to your app, multi-condition handling will keep the app scalable and maintainable if you are using React JS Includes Multi Condition.
Conclusion
React JS Includes Multi Condition is an important concept in React JS that provides you a lot of flexibility and efficiency in your applications. You can easily manage complex conditions by using ternary operator, logical AND (&&), switch-case, nested ternaries, and functions. This will keep your code clean, readable and maintainable.
If you are a React developer, these techniques will improve your coding practices and help you build more complex React applications.
If you found this blog useful, then do share your thoughts in the comments and if you want to know about more advanced concepts of React, then do not forget to follow our blog!
Also check it out our article.
3 thoughts on “React JS Includes Multi Condition”